SOFTWARE DESIGN PATTERNS
Spatial Partition
Spatial Partitioning is an optimization pattern meant to reduce the amount of individual distance calculations. Typically if you need to know the distinct between game objects for some reason like making the nearest AI enemy chase the player you would just put all of the enemies in a list and run distance calculations for all of them. This is okay if you were to only have a few but with a lot of actors in the scene these calculations can slow things down. Spatial Partitioning cashes the positions of these actors in a divided grid, so if your scene is a 4x4 grid and your player is in cell 2,3 only the actors in 2,3 care and run calculations.
This is a visualization of the spatial partition pattern to show the performance boost between the standard AI navigation and the spatial partition provided by a tutorial from Habradore which is linked in my Github repository. My modifications were changing the lighting effects, adding an on screen display of the update time, and a toggle for the spatial partition.

State Machine
The State Machine is a behavioral pattern which allows an actor to transition between different states with different functionalities and restrictions. For example if the user is not unputing any commands the actor will be in an idle state but if the user inputs jump command the actor will enter the jumping state and execute that behavior, then from jumping it can enter another state like diving but i can’t enter jumping again unless double jump is allowed.
This example is specifically a Finite State Machine because there is a set amount of available states the actor can enter and is a modification of a tutorial by Nystrom which is linked in my Github Repository.

Event Queue
An Event Queue is a decoupling pattern that allows multiple sources to fire off events and still have them recorded and executed in the proper order, first in first out. This can also be helpful if there is a noticeable delay because one or more events take longer to execute because any stored events will still execute in the proper order.
This example is a text based visualization based on a tutorial from Nystrom which is linked in my Github Repository. In this case different keys trigger events for a spaceship. The cannons firing and the self destruct both have a delay on them which will allow a backlog to build up and show the event queue working. I added in the accelerate and self district events.
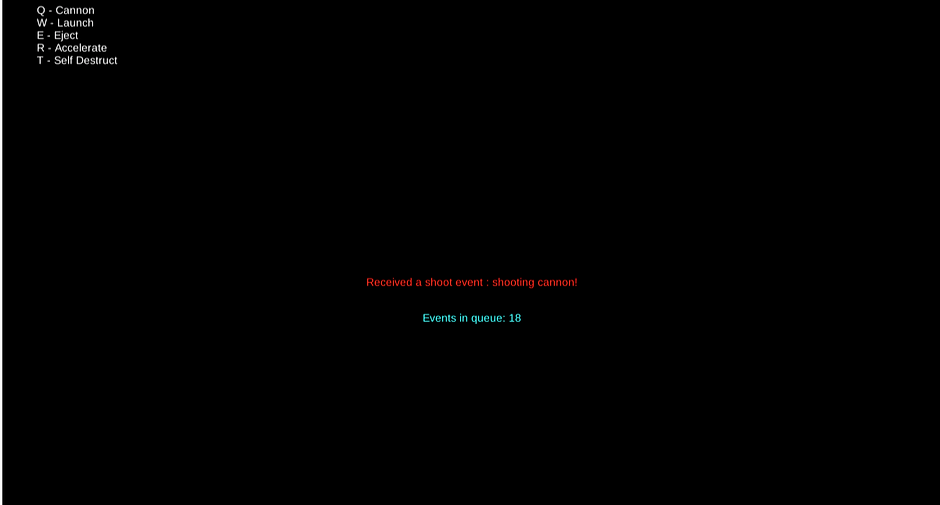
Decorator
The Decorator is a structural pattern which adds on new behaviors to a base object. When an object is created it can have behaviors from other classes added onto it in order to gain their behaviors which can allow for the fast creation of unique objects based on parameters or user input.
In this example the user is able to add different classes of adventurers to their party. This is a modification of the weapon customization tutorial from Baron that adds an infinite number of modifications to a base model. I made changes to the cosmetic and dramatic elements of this tutorial.
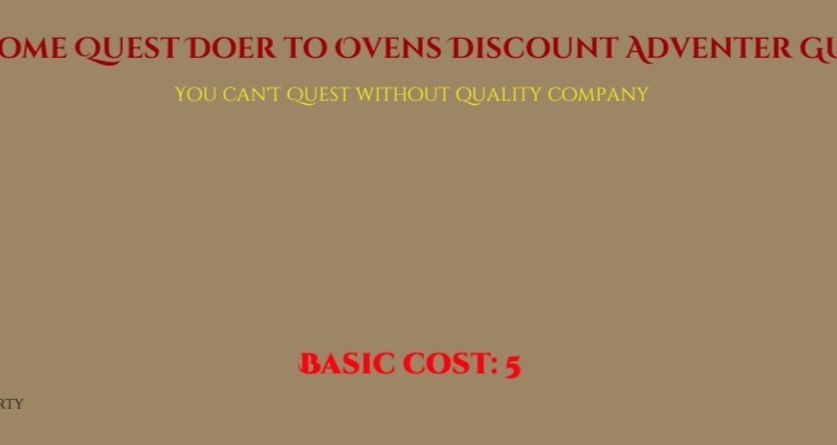
Abstract Factory
An Abstract Factory is a creational pattern that allows for the creation of new factories at runtime. A typical factory allows for new game objects or structures to be created on the fly based on parameters or user input. The abstract factory is a general factory that creates new more specific factories based on what is needed by the system.
This example is bestiary where you input the characteristics of a monster and the system will create the proper factory to output the monster you are describing. This comes from a modification of a tutorial for Baron. I improved the IU by making it dropdown based and importing low poly Maya assets that I made.

Observer
The Observer is a behavioral pattern that allows one system to notify another one that it has done something, which is very useful for adhering to the single responsibility principle. In general an observer is a system that is waiting for an event to be thrown from somewhere else and once it receives notice of the event the observer will execute whatever behavior it’s supposed to. It is worth noting that the system throwing the event does not care at all about whether or not an observer actually receives that notification.
This example is a modification of Cubethon from Brackeys and a tutorial from Baron both of which are linked in my Github Repository. I added in the words showing up on the sides, (Jean Wilder’s poem from Willy Wonka) based on distance traveled and two switches to turn on the obstacle lights and start them moving towards the player.
